Question 1 (a): Write the name of weightSum and layout_weight attribute used in the Linear layout.
In Android’s Linear Layout, there are two attributes that define the weight of a view, which are:
android:layout_weight
android:weightSum
Question 1 (b): The layout manager provides common attribute layout height for views. Is there any difference between the match parent and Odp value used for these attributes?
Yes, there is a difference between “match_parent” and “odp” (or “fill_parent”) values used for the layout height attribute in Android.
“match_parent” is used to specify that the view should be as tall as its parent layout, taking up all the available vertical space. This means that the height of the view will be determined by the height of the parent layout.
On the other hand, “odp” or “fill_parent” is also used to specify that the view should take up all the available vertical space in the parent layout. However, this value is deprecated since API level 8 and should be avoided.
Question 1 (c): Which method is used to register a context menu to a ListView item? Explain Briefly.
The registerForContextMenu()
method is used to register a context menu to a ListView item in Android. This method is called on the ListView object and takes a parameter that is the View for which the context menu should be registered.
Here’s a brief explanation of how this method works:
- First, you need to create a context menu resource file which defines the menu items that will appear when a user long-presses on a ListView item.
- Then, in your Activity or Fragment that contains the ListView, you override the
onCreateContextMenu()
method which is called when the user performs a long press on an item in the ListView. - Inside the
onCreateContextMenu()
method, you inflate the context menu resource file using theMenuInflater
and then add it to the context menu using theadd()
method. - Finally, you call the
registerForContextMenu()
method on the ListView object passing in the View for which the context menu should be registered.
Question 1 (d): Briefly explain the terms density-independent pixel (DP) and Scale independent pixel (sp)
Density-independent pixels (DP) is a unit of measurement used to define lengths and sizes in a way that is independent of the device’s display density. DP is based on a virtual density of 160 dots per inch (dpi), which is approximately the average density of a medium-density screen. By using DP, developers can define UI elements in a way that will look consistent across different devices, regardless of their screen density. For example, an image defined in 100DP will look the same size on a high-density screen and a low-density screen.
Scale-independent pixels (sp) is a unit of measurement used for text size, which is also density-independent but scaled according to the user’s font size preference. SP is like DP, but it also takes into account the user’s font size preference. By using SP, developers can define text size that will look consistent across different devices and also adjust according to the user’s font size preference. For example, if the user increases their font size preference, the text defined in 16sp will appear larger, but the ratio between the text size and other UI elements defined in DP will remain the same.
Question 1 (e): Write Java code for the following:
(i) Array adapter
(ii) Popup Menu
(i) Array adapter
String[] items = {"Item 1", "Item 2", "Item 3"}; ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, items); ListView listView = findViewById(R.id.list_view); listView.setAdapter(adapter);
(ii) Popup Menu
Button button = findViewById(R.id.button); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { PopupMenu popupMenu = new PopupMenu(MainActivity.this, button); popupMenu.getMenuInflater().inflate(R.menu.popup_menu, popupMenu.getMenu()); popupMenu.setOnMenuItemClickListener(new PopupMenu.OnMenuItemClickListener() { @Override public boolean onMenuItemClick(MenuItem item) { switch (item.getItemId()) { case R.id.option1: // do something for option 1 return true; case R.id.option2: // do something for option 2 return true; default: return false; } } }); popupMenu.show(); } });
Question 2 (a): What do you understand by the term Android Activity stack? Explain any two types of launch modes available in Android, excluding the standard launch mode.
In Android, an activity stack is a collection of activities that are arranged in a stack format. It is a data structure that keeps track of the current state of all the activities that are running in an application. The activity on top of the stack is the one that the user is currently interacting with.
Two types of launch modes available in Android, excluding the standard launch mode are:
- SingleTop: When a new instance of an activity is about to be started and if the top of the activity stack already contains an instance of the same activity, then instead of creating a new instance of the activity, the existing instance is brought to the top of the stack and its onNewIntent() method is called. This is useful when an application wants to handle a new intent for an existing activity instance.
- SingleTask: In this mode, the system creates a new task and a new instance of the activity on top of the task if there is no instance of the activity running in the system. If an instance of the activity already exists in a task, then the entire task is brought to the foreground and its onNewIntent() method is called. This is useful when an application wants to maintain a separate stack for a particular activity.
Question 2 (b): What do you understand by the term instance state of activity? Write Java code to explain how it can be saved and restored. Use appropriate lifecycle methods to support your answer.
In Android, an activity’s instance state is the collection of values that define the activity’s state at a given time. This includes things like user input, current data, and other variables that may change over time. To ensure that an activity can be restored to its previous state after being destroyed and recreated, it is important to save and restore the instance state.
public class MainActivity extends AppCompatActivity { private int counter = 0; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); if (savedInstanceState != null) { counter = savedInstanceState.getInt("counter"); } Button btnIncrement = findViewById(R.id.btn_increment); final TextView tvCounter = findViewById(R.id.tv_counter); btnIncrement.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { counter++; tvCounter.setText(String.valueOf(counter)); } }); } @Override protected void onSaveInstanceState(Bundle outState) { super.onSaveInstanceState(outState); outState.putInt("counter", counter); } @Override protected void onRestoreInstanceState(Bundle savedInstanceState) { super.onRestoreInstanceState(savedInstanceState); counter = savedInstanceState.getInt("counter"); } }
Question 3: Write a Java class of your choice which should have at least three data members, one for the data type Integer and two Strings. Write Java code to initialize this class instance. Send this class instance using a parcel to another activity on click of a submit button.
public class MyClass implements Parcelable { private int myInt; private String myString1; private String myString2; public MyClass(int myInt, String myString1, String myString2) { this.myInt = myInt; this.myString1 = myString1; this.myString2 = myString2; } public int getMyInt() { return myInt; } public void setMyInt(int myInt) { this.myInt = myInt; } public String getMyString1() { return myString1; } public void setMyString1(String myString1) { this.myString1 = myString1; } public String getMyString2() { return myString2; } public void setMyString2(String myString2) { this.myString2 = myString2; } @Override public int describeContents() { return 0; } @Override public void writeToParcel(Parcel dest, int flags) { dest.writeInt(myInt); dest.writeString(myString1); dest.writeString(myString2); } public static final Parcelable.Creator<MyClass> CREATOR = new Parcelable.Creator<MyClass>() { @Override public MyClass createFromParcel(Parcel in) { return new MyClass(in); } @Override public MyClass[] newArray(int size) { return new MyClass[size]; } }; private MyClass(Parcel in) { myInt = in.readInt(); myString1 = in.readString(); myString2 = in.readString(); } }
Question 4: Write Java code for an Android application to trigger a notification for Android SDK versions lower than Oreo with the following details:
(i) Channel ID: ANDROID_EXAM CHANNEL
(ii) Content Title: Broadcast Notification
(iii) Content Text: Hello
// Import required packages import android.app.NotificationManager; import android.app.PendingIntent; import android.content.Context; import android.content.Intent; import android.support.v4.app.NotificationCompat; public class NotificationUtils { private static final String CHANNEL_ID = "ANDROID_EXAM CHANNEL"; public static void sendNotification(Context context) { // Create an intent to open the app when the notification is clicked Intent intent = new Intent(context, MainActivity.class); PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT); // Create a notification builder and set its properties NotificationCompat.Builder builder = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("Broadcast Notification") .setContentText("Hello") .setContentIntent(pendingIntent) .setPriority(NotificationCompat.PRIORITY_DEFAULT); // Get the notification manager and send the notification NotificationManager notificationManager = (NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE); notificationManager.notify(0, builder.build()); } }
Question 5: How do you return results from an activity? Write Java code for implementing the same.
In Android, you can return results from an activity to the calling activity using the setResult()
method and the onActivityResult()
method.
// In the calling activity public static final int REQUEST_CODE = 1; // Start the second activity Intent intent = new Intent(this, SecondActivity.class); startActivityForResult(intent, REQUEST_CODE); // Handle the result from the second activity @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == REQUEST_CODE) { if (resultCode == RESULT_OK) { String result = data.getStringExtra("result"); Toast.makeText(this, "Result: " + result, Toast.LENGTH_SHORT).show(); } else if (resultCode == RESULT_CANCELED) { Toast.makeText(this, "Cancelled", Toast.LENGTH_SHORT).show(); } } } // In the second activity // Return the result to the calling activity Intent intent = new Intent(); intent.putExtra("result", "Hello World"); setResult(RESULT_OK, intent); finish();
Question 6: Write Java code for an Android activity that contains a button. On click of this button trigger a numeric counter from 0 to 100, on a new thread of an unable interface. The counter should increment the value after every 250 milliseconds.
import android.os.Bundle; import android.os.Handler; import android.os.Looper; import android.view.View; import android.widget.Button; import android.widget.TextView; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { private Button startButton; private TextView counterText; private int counter = 0; private Handler handler = new Handler(Looper.getMainLooper()); private Runnable counterRunnable = new Runnable() { @Override public void run() { counterText.setText(String.valueOf(counter)); if (counter < 100) { counter++; handler.postDelayed(this, 250); } } }; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); startButton = findViewById(R.id.start_button); counterText = findViewById(R.id.counter_text); startButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { counter = 0; handler.post(counterRunnable); } }); } }
Question 7: Write Java code to create an Android application for the given layout, which uses the Contacts content provider to fetch the phone no of user “AMIT” from the contacts and display it on a TextView. Display “No record found” if no such contact exists.
(Note: for API 23 and above runtime permission is required)
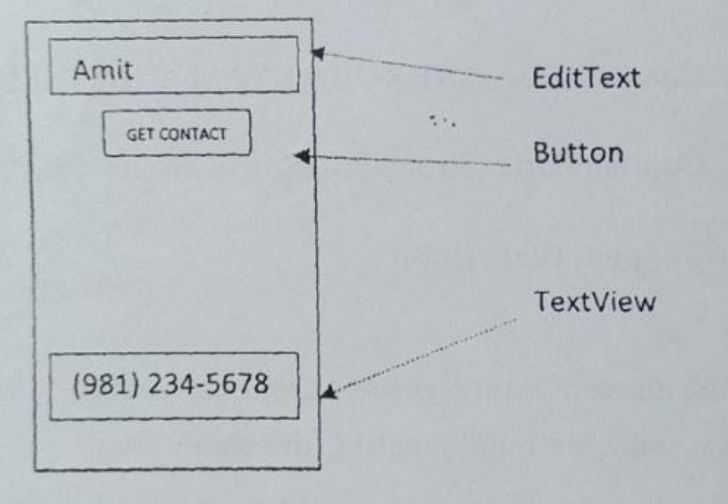